API Integration can seem a bit daunting, but trust me, it’s one of the most powerful tools in modern web development. Today we’re going to explore API Integration, from its basic working mechanism to its importance, and then see how HTML APIs and Microformats can make your web projects smarter and more interactive, of course, real-world examples will connect all the dots. Ready? Let’s dive in!🚀
Table of Contents
What is an API?
- API stands for (Application Programming Interface).
- An API is a set of protocols and tools that allow two software systems to communicate.
- It acts as an intermediary, defining methods and data structures for interactions.
- Developers use APIs to request and send data between services.
- APIs allow interaction without needing to understand the internal code or design of the other system.
- An API can be compared to a waiter in a restaurant: it takes your order (data request) and brings back the food (response) from the kitchen (server).
What is API Integration?
API integration is all about making your web applications talk to other systems. Think of APIs (Application Programming Interfaces) as messengers between different platforms—letting them exchange data or trigger functions behind the scenes.
Let’s say you have a Weather App on your website. One way to showcase the latest data is to build your entire weather forecasting system, but is that even easy, so why not just integrate a weather API, and BOOM, your work is done. Now you can showcase the latest weather data on your website. This is what APIs do.
Why is API Integration Becoming a Standard?
Here are the key reasons API integration is now a standard practice in web development:
- Faster Development: APIs allow developers to integrate existing features quickly, reducing development time.
- Seamless Data Sharing: APIs enable smooth and easy data exchange between different platforms.
- Real-Time Functionality: They provide up-to-date information, allowing apps to update instantly with real-time data.
- Scalability: APIs make it easier to expand and add new services without changing the entire system.
- Cost-Effective: Using APIs reduces the need for custom-built features, cutting down development costs.
- Enhanced User Experience: APIs create dynamic, interactive features that improve overall user engagement.
- Flexibility: APIs allow apps to connect with multiple services, offering broader functionality and integration options.
Benefits of API Integration:
- Efficiency: You do not need to reinvent the wheel. APIs let you use existing functionalities.
- Time-Saving: It takes no time to integrate third-party services.
- Enhanced User Experience: APIs make websites dynamic and interactive, leading to richer user experiences.
Drawbacks and Challenges:
- Compatibility Issues: APIs do not sometimes play nice with various browsers or devices.
- Security Risks: Since APIs usually connect with third-party services, they introduce the risk of vulnerabilities if not secured appropriately.
- Performance Impact: A slow API affects your site speed, which hurts the user experience of the site.
Real-World Example: Integrating the Geolocation API
Let’s walk through a simple example with the Geolocation API—a built-in HTML API that fetches a user’s location.
Step-by-Step Guide to Integrating the Geolocation API:
- Step 1: Check if the user’s browser supports geolocation.
- Step 2: Request the user’s permission to access their location.
- Step 3: Use the latitude and longitude data returned by the API to display their location.
Here’s a quick example:
<button onclick="getLocation()">Get Location</button> <p id="location"></p> <script> function getLocation() { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(showPosition); } else { document.getElementById("location").innerHTML = "Geolocation not supported."; } } function showPosition(position) { document.getElementById("location").innerHTML = "Latitude: " + position.coords.latitude + "<br>Longitude: " + position.coords.longitude; } </script>
This is just integration, To make it truly beautiful let’s do some CSS.
<style> body { font-family: Arial, sans-serif; background-color: #c6e5ef; display: flex; justify-content: center; align-items: center; flex-direction: column; height: 100vh; margin: 0; } button { background-color: #4CAF50; color: #fff; padding: 10px 20px; border: none; border-radius: 5px; cursor: pointer; } button:hover { background-color: #3e8e41; } #location { font-size: 18px; margin-top: 20px; padding: 10px; background-color: #c6e5ef; border: 1px solid #ddd; border-radius: 5px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); text-align: center; } #location span { display: block; margin-bottom: 10px; } </style> <button onclick="getLocation()">Get Location</button> <div id="location"></div> <script> function getLocation() { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(showPosition); } else { document.getElementById("location").innerHTML = "Geolocation not supported."; } } function showPosition(position) { document.getElementById("location").innerHTML = "<span>Latitude: " + position.coords.latitude + "</span><br><span>Longitude: " + position.coords.longitude + "</span>"; } </script>
Output:
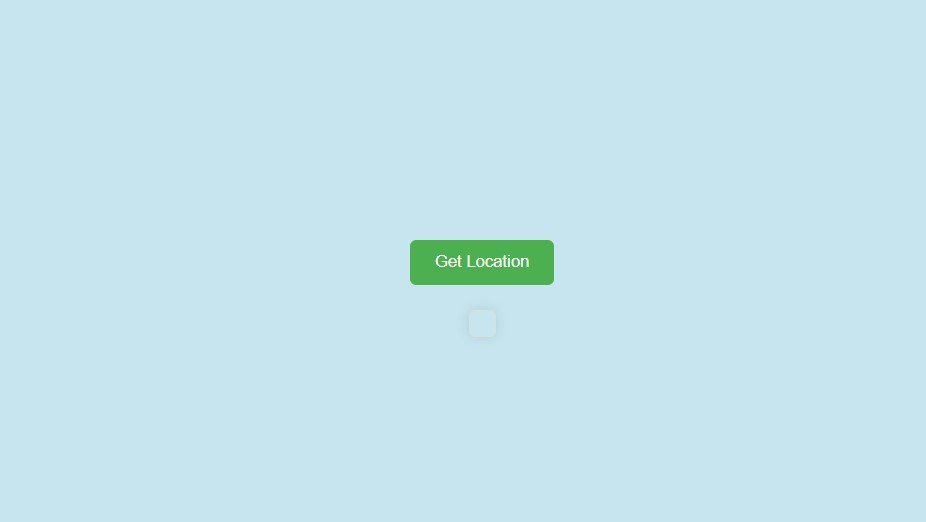
Location:

What just happened?
When the user clicks the button, the browser asks for permission to access their location. If they agree, the API returns their latitude and longitude. Simple and effective!
Common Pitfalls:
- User Denial: If the user denies location access, your app needs to handle that gracefully (like showing a default location).
- Device Compatibility: Always test on multiple devices because some older browsers may not support certain APIs.
Also Read:
- How to Connect Two Pages of a Website: The Ultimate Guide
- The Ultimate HTML Cheat Sheet: Code Like a Pro!
- HTML Tables Tutorial with Examples: Become An Expert In 15 Minutes
- Learn More about web APIs.
Quiz:
API Integration and Microformats Quiz
What Are Microformats?
Now let’s talk about Microformats. These are not APIs, but these are HTML-based patterns, providing extra meaning to your web content. Consider them as tiny markers helping search engines, browsers, and other apps understand the structure of your data – thereby boosting your SEO and enhancing accessibility.
Benefits of Using Microformats:
- SEO Boost: Microformats make it easier for search engines to read and rank your content.
- Enhanced Usability: They help browsers or external apps extract useful information from your webpage, such as contact details or reviews.
- Accessibility: Microformats can improve the user experience for screen readers and other assistive technologies.
Real-World Example: Integrating hCard for Contact Information
The hCard Microformat is used for marking up contact information. Here’s a simple example of how to integrate it:
<div class="hcard"> <h1 class="fn">Areeb Anwar</h1> <div class="org">WebDevTales</div> <a class="url" href="https://webdevtales.com">webdevtales.com</a> <div class="tel">+1 (123) 456-7890</div> <div class="email"><a href="mailto:jane.doe@example.com">jane.doe@example.com</a></div> <div class="adr"> <div class="street-address">Address: 123 Main St</div> <div class="locality">Locality: Anytown</div> <div class="region">Region: CA</div> <div class="postal-code">Postal Code: 12345</div> <div class="country-name">Country Name: USA</div> </div> </div> <!-- Little CSS TO MAKE it beautiful to eye. --> <style> /* Global Styles */ * { box-sizing: border-box; margin: 0; padding: 0; } body { font-family: Arial, sans-serif; line-height: 1.6; color: #333; background-color: #f9f9f9; } /* hCard Styles */ .hcard { max-width: 300px; margin: 40px auto; padding: 20px; background-color: #fff; border: 1px solid #ddd; border-radius: 10px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); display: flex; flex-direction: column; align-items: center; } .fn { font-size: 24px; font-weight: bold; margin-bottom: 10px; color: #337ab7; } .org { font-size: 18px; color: #666; margin-bottom: 20px; } .url { font-size: 16px; color: #337ab7; text-decoration: none; transition: color 0.2s ease; } .url:hover { color: #23527c; } .tel { font-size: 16px; color: #337ab7; margin-bottom: 20px; } .email { font-size: 16px; color: #337ab7; margin-bottom: 20px; } .adr { margin-bottom: 20px; display: flex; flex-direction: column; align-items: center; } .street-address { font-size: 16px; margin-bottom: 10px; } .locality { font-size: 16px; margin-bottom: 10px; } .region { font-size: 16px; margin-bottom: 10px; } .postal-code { font-size: 16px; margin-bottom: 10px; } .country-name { font-size: 16px; margin-bottom: 20px; } </style>
Output:

In this example, the hCard format helps search engines recognize the text as contact information. This could boost your site’s visibility in local search results.
Trends and Challenges in API Integration and Microformats
Trends in API Integration:
- Increased Use of Real-Time Data: Applications such as chat systems use APIs like WebSockets for the exchange of real-time data.
- API-First Development: More companies are prioritizing API development to ensure their services can be easily integrated with other systems.
Challenges with APIs:
- Over-reliance on Third-Party APIs: The functionality of your website is hindered when the service you rely on is down.
- API Versioning: Some APIs change over time and your app will need to adapt to keep track of such updates.
Trends in Microformats:
- Structured Data for SEO: Google and other search engines are increasingly relying on structured data like Microformats to provide rich search results.
- Schema.org: Microformats are being used alongside or in place of schema.org for marking up content.
HTML APIs
Here’s a list of notable HTML APIs:
- WebRTC API – Enables real-time communication for audio and video.
- Geolocation API – Provides access to the geographical location of a device.
- Web Storage API – Allows storage of data in the browser, including localStorage and sessionStorage.
- Canvas API – Enables drawing and rendering graphics on the web.
- Web Workers API – Allows running scripts in the background, separate from the main thread.
- Fetch API – Provides an interface for making HTTP requests to fetch resources.
- Web Sockets API – Enables real-time communication between the server and client.
- Media Capture API – Allows access to media streams, such as video and audio, from devices.
- File API – Provides the ability to read and manipulate files stored on the client’s device.
- Notification API – Allows web pages to send notifications to users.
- Service Workers API – Enables background processing for offline capabilities and caching.
- Drag and Drop API – Facilitates drag-and-drop interactions for user interfaces.
- History API – Allows manipulation of the browser history, enabling navigation without page refresh.
- Speech Recognition API – Provides functionality for recognizing and processing voice input.
- Pointer Events API – Offers a unified way to handle mouse, touch, and stylus events.
Conclusion: The Future of API Integration and Microformats
Integrating APIs and Microformats into your web projects opens up a world of possibilities. By leveraging these tools, you can enhance the user experience, boost your SEO, and speed up development. While there are some challenges, the benefits far outweigh the drawbacks.
Stay tuned! Step-by-step guides for integrating various HTML APIs, like Web Storage, WebRTC, and more, will be coming soon to help you master API integration one API at a time.
Happy coding! 👩💻👨💻
FAQs:
What is an API?
An API (Application Programming Interface) is a set of protocols that allows different software systems to communicate with each other and share data or functions.
Why is API integration important?
API integration allows applications to use third-party services, enabling faster development, real-time data updates, and enhanced functionality without needing to build everything from scratch.
What are the common challenges of API integration?
Common challenges include compatibility issues, security risks, versioning problems, and handling performance impacts if the API is slow or unavailable.
Can APIs be used on all websites?
Yes, APIs can be integrated into almost any website or web application, but compatibility may vary depending on the platform and the specific API being used.
What is the difference between an API and a microservice?
An API is a method of communication between systems, while a microservice is an architectural style where applications are built as a collection of smaller services. APIs are often used to connect these microservices.